What is Cookies in Angular?
Cookies are small packages of information that can be temporarily stored/saved by your browser and websites which are using cookies for multiple things.
Cookies are used in multiple requests and browser sessions and can store your account information used by authentication for example.
Also Read: Transpilation in Angular
Size of Cookies
They are often not more than a few kilobytes per cookie.
How to install Cookies Dependency
We already have an NPM package for Angular called ‘ngx-cookie-service‘ that can be used for cookie use.
Let’s install the cookies dependency using below command:
2 3 4 |
npm install ngx-cookie-service |
After installing the cookies dependency, we have to import the CookieService
inside one of our modules and add them as a provider. Please refer the below example code:
app.module.ts file
2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; @NgModule({ declarations: [AppComponent], imports: [BrowserModule], providers: [CookieService], bootstrap: [AppComponent] }) export class AppModule { } |
Also Read: Angular Online Quiz Test
How to use Cookies in Angular
Now we will use our AppComponent and use the set, get and delete method of the CookieService. In the below example code,
app.component.ts file
2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import { Component} from '@angular/core'; import {CookieService} from 'ngx-cookie-service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], }) export class AppComponent { private cookie_name=''; private all_cookies:any=''; constructor(private cookieService:CookieService){ } setCookie(){ this.cookieService.set('name','Tutorialswebsite'); } deleteCookie(){ this.cookieService.delete('name'); } deleteAll(){ this.cookieService.deleteAll(); } ngOnInit(): void { this.cookie_name=this.cookieService.get('name'); this.all_cookies=this.cookieService.getAll(); // get all cookies object } } |
we import the CookieService inside the app.component.ts file.
2 3 4 |
import { CookieService } from 'ngx-cookie-service'; |
Next step, we will inject this service in the parameters of the constructor and set the private variable.
2 3 4 5 |
constructor( private cookieService: CookieService ) { } |
Set, get and getAll Cookies
In the first line, we are using set function to set the new cookie value with name. In the second line, we are using get function to get the cookie value with cookie name.
2 3 4 5 6 |
this.cookieService.set('name','Tutorialswebsite'); // set the cookies value this.cookie_name=this.cookieService.get('name'); // get the cookie value this.all_cookies=this.cookie.getAll(); // get All cookies object |
Delete and Delete All Cookies
In the first line, we are using delete function to delete the single cookie value with name. In the second line, we are using deleteAll function to delete all cookie value with single click.
2 3 4 5 |
this.cookieService.delete('name'); // delete the single cookies value this.cookieService.deleteAll(); // delete all the cookie value |
app.component.html file
We use the below code to display the output in browser and trigger set Cookies, Delete and Delete All function.
2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<div style="text-align: center;" > <h5>Cookies In Angular</h5> <h5>Cookie Data:</h5> <p>Name: {{cookie_name}}</p> <p>Cookie Object: {{all_cookies | json}}</p> <br> <button (click)="setCookie()">Set Cookies</button> <br><br> <button (click)="deleteCookie()">Delete</button> <br><br> <button (click)="deleteAll()">Delete All</button> </div> |
Method of Cookies in Angular
- Check :- Used to check cookies exits or not
- Set :- Used to set the value in cookies with name
- Get :- Used to return the single value of stored cookies name
- Get All :- Used to return a value object of all cookies
- Delete :- Used to delete the single cookies value with the given name
- Delete All :- Used to delete all the cookies
Conclusion
Thank you for reading How to use cookies in angular article. Hope I was able to help someone out. If you have any questions feel free to ask anything on the comment section. Cheers!!.
Are you want to get implementation help, or modify or extend the functionality of this script? Submit a paid service request
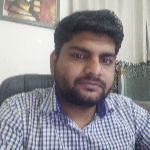
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website”. He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone, e27.co